November
TOP 10 PHP MySQL Web Development Security Tips
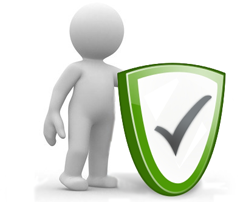
Here is the some security tips for PHP MYSQL web development tips. Checkout the below points, it would be very useful for in developing secure web application.
If you are expecting an integer call intval() (or use cast) or if you don’t expect a username to have a dash (-) in it,
check it with strstr() and prompt the user that this username is not valid.
Here is an example:
PHP Code:
$post_id = intval($_GET[‘post_id’]);
mysql_query(“SELECT * FROM post WHERE id = $post_id”);
Now $post_id will be an integer for sure
2. Validate user input on the server side
If you are validating user input with JavaScript, be sure to do it on the server side too, because for bypassing your
JavaScript validation a user just needs to turn their JavaScript off.
JavaScript validation is only good to reduce the server load.
3. Do not use user input directly in your SQL queries
Use mysql_real_escape_string() to escape the user input.
PHP.net recommends this function: (well a little different)
PHP Code:
function escape($values) {
if(is_array($values)) {
$values = array_map(array(&$this, ‘escape’), $values);
} else {
/* Quote if not integer */
if ( !is_numeric($values) || $values{0} == ’0? ) {
$values = “‘” .mysql_real_escape_string($values) . “‘”;
}
}
return $values;
}
Then you can use it like this:
PHP Code:
$username = escape($_POST[‘username’]);
mysql_query(“SELECT * FROM user WHERE username = $username”); /* escape() will also adds quotes to strings automatically */
4. In your SQL queries don’t put integers in quotes
For example $id is suppose to be an integer:
PHP Code:
$id = “0; DELETE FROM users”;
$id = mysql_real_escape_string($id); // 0; DELETE FROM users – mysql_real_escape_string doesn’t escape ;
mysql_query(“SELECT * FROM users WHERE id=’$id’”);
Note that, using intval() would fix the problem here.
5. When uploading files, validate the file mime type
If you are expecting images, make sure the file you are receiving is an image or it might be a PHP script that can run
on your server and does whatever damage you can imagine.
One quick way is to check the file extension:
PHP Code:
$valid_extensions = array(‘jpg’, ‘gif’, ‘png’); // …
$file_name = basename($_FILES[‘userfile’][‘name’]);
$_file_name = explode(‘.’, $file_name);
$ext = $_file_name[ count($_file_name) – 1 ];
if( !in_array($ext, $valid_extensions) ) {
/* This file is invalid */
}
6. Give your database users just enough permissions
If a database user is never going to drop tables, then when creating that user don’t give it drop table permissions,
normally just SELECT, UPDATE, DELETE, INSERT should be enough.
7. Do not allow hosts other than localhost to connect to your database
If you need to, add only that particular host or IP as necessary but never, ever let everyone connect to your database server.
8. Your library file extensions should be PHP
.inc files will be written to the browser just like text files (unless your server is setup to interpret them as PHP scripts),
users will be able to see your messy code (kidding) and possibly find exploits or see your passwords etc.
Have extensions like config.inc.php or have a .htaccess file in your extension (templates, libs etc.) folders with this one line:
Code:
deny from all
9. Have register globals off or define your variables first
Register globals can be very dangerous, consider this bit of code:
PHP Code:
if( user_logged_in() ) {
$auth = true;
}
if( $auth ) {
/* Do some admin stuff */
}
If you have registered globals on and you can’t turn it off for some reason you can fix these issues by defining your variables first:
PHP Code:
$auth = false;
if( user_logged_in() ) {
$auth = true;
}
if( $auth ) {
/* Do some admin stuff */
}
Defining your variables first is a good programming practice that I suggest you follow anyway.
10. Keep PHP itself up to date
Just take a look at www.php.net and see release announcements and note how many security issues they
fix on every release to understand why this is important.